Abstract Class and Abstract Method in Python Programming
Abstract Class: Abstract class in python programming is the class that contains one or more abstract methods. These types of classes in python are called abstract classes. Abstract methods are the methods that have an empty body or we can say that abstract methods have the only declaration but it doesn’t have any functional implementation.
Important: Abstract classes cannot be instantiated directly. We need subclass inheriting abstract class to instantiate. But before creating the object or instantiating the subclass we need to provide implementations for abstract methods.
Flow Diagram For Abstract Class
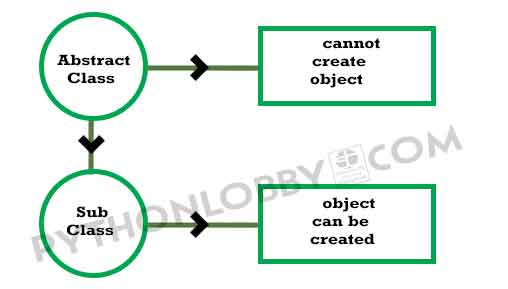
Syntax:
Above image perfectly shows the syntax and syntax explanation of how to create abstract class and methods.
Explanation: Here we have imported ABC class from pre-defined abc library. Also we have imported abstract method decorator. We will discuss about decorators later.
We have defined the class Test which is inheriting ABC class that we have imported from abc library. Inside Test class, two abstract methods are declared (method1_name and method2_name). This complete class is called abstract class. Now we see one example to understand it in more detail.
Example 1: According to statement, we cannot directly instantiate or create object of abstract class. Let’s see by doing this. What happen if we create object of abstract class:
# abstract_class_example from abc import ABC, abstractmethod class Test(ABC): @abstractmethod def ex(self): None obj = Test()
Output:
# Output # TypeError: Can't instantiate abstract class Test with abstract methods ex
We created the object of abstract class directly, hence it returned an error.
Example 2: According to statement, abstract class can only be instantiated by using its subclass, and abstract method declared in parent abstract class should be defined in its subclass. Let’s see by doing.
# abstract_class_example from abc import ABC, abstractmethod class A(ABC): @abstractmethod def ex(self): None class Test(A): # abstractmethod defined in child or Subclass def ex(self): print("Method defined in Subclass") obj = Test() obj.ex()
Output:
# Output # Method defined in Subclass
Hence it works fine……
I don’t want to make it more complex. That’s all about the abstract classes and abstract methods in python.
I hope it is enough for clear understanding about abstract class and abstract methods.