Polymorphism in Python Programming
Polymorphism in Python: Polymorphism means different or many forms. The word polymorphism is taken from the Greek word poly (many) morphisms (forms). There are also several types of polymorphism in python.
We all know a child class can inherit the properties of parent class including class methods. In some cases, the methods inherited from parent class are not sufficient. So, in that case we re-implement those methods in child class. The process of modifying or adding more features to already defined method is called Polymorphism in python.
The main difference between overloading and overriding is that overloading is the concept in which same function with same name and different parameters are used for different purposes. This is called method overloading. And overriding follows the rule of having the same method name with same parameters. This is called method overriding.
Types of Polymorphism in Python Programming
Types of Polymorphism:
1). Operator Overloading
2). Method Overriding in Inheritance (user-defined)
3). Method Overriding (in-built)
4). Method Overloading
(i). Operator Overloading in python: We all know that plus (+) operator in python is used for the addition of elements in mathematics. But it is also used for other operations also. The process of using same operator for different use is called Operator Overloading.
Example 1:
#Example_operator_overloading class Abc: x = 2+3 y = "raj"+"aryan" obj = Abc() print(obj.x) print(obj.y)
Output:
#Output PythonLobby.com
Hence, we used same plus(+) operator for different purposes. One for mathematical addition and second for string concatenation.
(ii). Method Overriding (Inheritance): When we have method defined in parent class and also in child class with same parameter and same method name. To enhance the functionality of method in child class is called method overriding in python. See below given example to understand the concept of method overriding.
Example 1:
#Example_method_overriding class Abc: def fncn(self): print("Parent class") class xyz(Abc): def fncn(self): print("Child class") obj = xyz() obj.fncn()
Output:
#Output Child class
In above example, we have defined fncn() method in parent class and then inherited the properties of parent class in child class. Later we found that the method defined in parent class is not sufficient, so we override that method.
(iii). Method Overriding (in inbuilt – functions): There are some inbuilt methods of python that are used or satisfy the rule of method overriding. We are talking about len() method.
Example 2:
#Example_operator_overriding x_string = "pythonlobby.com" x_list = [1,2,3,4,5] x_dict = {0:'P',1:'y',2:'t',3:'h',4:'o',5:'n'} print(len(x_string)) print(len(x_list)) print(len(x_dict))
Output:
#Output 15 5 6
So, we easily observe that len() method is working fine with all of our above data type. But it returning different output for different entity.
Diagram to elaborate the len() function in detail
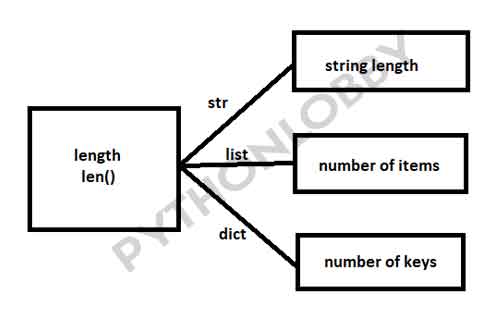
4). Method Overloading: In other programming languages, like java, C and C++ method overloading concept is supported but in Python method overloading is not supported.